How can I efficiently loop through opportunities on a list of accounts?
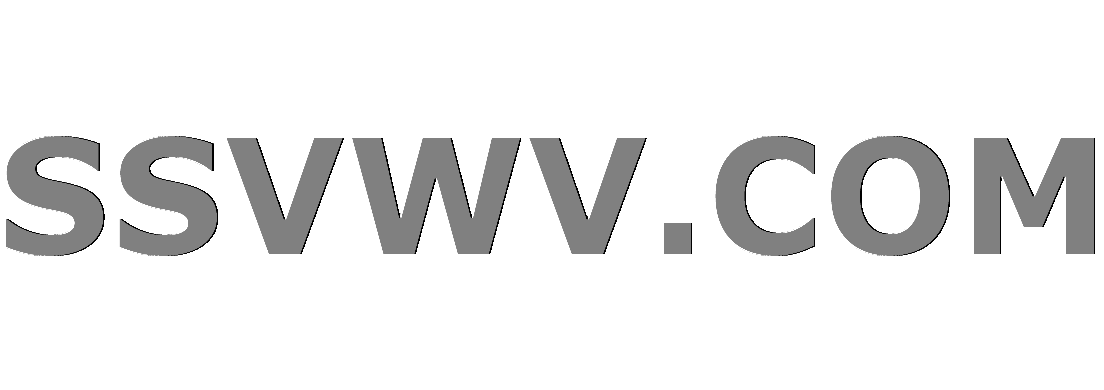
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ margin-bottom:0;
}
Currently we have a few nested for loops in our codebase that are doing what is mentioned in the question, but if we data load a large amount of records we run into CPU time errors. I am wanting to optimize as much as possible by eliminating these nested for loops. How can I perform operations on each opportunity in a list of accounts efficiently? An example of the pattern I'm trying to change is below.
List<account> accounts = [
SELECT id, Name, (SELECT id, StageName, CloseDate, OwnerId from Opportunities)
FROM Account
WHERE id IN :accountIds
];
for (Account acc : accounts) {
for (opportunity opp : acc.opportunities) {
//Opportunity operations here;
}
} //Want to optimize this logic
apex performance loop
add a comment |
Currently we have a few nested for loops in our codebase that are doing what is mentioned in the question, but if we data load a large amount of records we run into CPU time errors. I am wanting to optimize as much as possible by eliminating these nested for loops. How can I perform operations on each opportunity in a list of accounts efficiently? An example of the pattern I'm trying to change is below.
List<account> accounts = [
SELECT id, Name, (SELECT id, StageName, CloseDate, OwnerId from Opportunities)
FROM Account
WHERE id IN :accountIds
];
for (Account acc : accounts) {
for (opportunity opp : acc.opportunities) {
//Opportunity operations here;
}
} //Want to optimize this logic
apex performance loop
add a comment |
Currently we have a few nested for loops in our codebase that are doing what is mentioned in the question, but if we data load a large amount of records we run into CPU time errors. I am wanting to optimize as much as possible by eliminating these nested for loops. How can I perform operations on each opportunity in a list of accounts efficiently? An example of the pattern I'm trying to change is below.
List<account> accounts = [
SELECT id, Name, (SELECT id, StageName, CloseDate, OwnerId from Opportunities)
FROM Account
WHERE id IN :accountIds
];
for (Account acc : accounts) {
for (opportunity opp : acc.opportunities) {
//Opportunity operations here;
}
} //Want to optimize this logic
apex performance loop
Currently we have a few nested for loops in our codebase that are doing what is mentioned in the question, but if we data load a large amount of records we run into CPU time errors. I am wanting to optimize as much as possible by eliminating these nested for loops. How can I perform operations on each opportunity in a list of accounts efficiently? An example of the pattern I'm trying to change is below.
List<account> accounts = [
SELECT id, Name, (SELECT id, StageName, CloseDate, OwnerId from Opportunities)
FROM Account
WHERE id IN :accountIds
];
for (Account acc : accounts) {
for (opportunity opp : acc.opportunities) {
//Opportunity operations here;
}
} //Want to optimize this logic
apex performance loop
apex performance loop
edited Feb 14 at 18:16


Adrian Larson♦
110k19121259
110k19121259
asked Feb 14 at 18:14


JoshJosh
22211
22211
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Nested loops are not inherently evil, and are required if you're working on a nested structure like this.
You could rewrite this particular query to be on Opportunity
instead of Account
, but CPU limit issues usually run deeper than a simple nested loop or two.
About the only answer I can give you here is that you need to spend a fair amount of time profiling your code so you can accurately characterize the overall performance, and identify the problem areas (which methods are being called most often? which methods consume the most CPU time/queries/etc...?)
Turning up the debug log levels for the Apex category, and making use of the "Analysis" perspective in the dev console (With a debug log open in the dev console: Test -> Switch Perspective -> Analysis) will give you additional buttons and panels to play around with that should help you gather more information.
add a comment |
You could try querying the Opportunity table like so.
List<Opportunity> opp = [Select Id From Opportunity Where
AccountId IN (Select Id From Account Where Id = :accountIds)];
Otherwise if you are running into heap errors you might need to optimize your code before and after the query or move your code into batch.
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "459"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsalesforce.stackexchange.com%2fquestions%2f250387%2fhow-can-i-efficiently-loop-through-opportunities-on-a-list-of-accounts%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Nested loops are not inherently evil, and are required if you're working on a nested structure like this.
You could rewrite this particular query to be on Opportunity
instead of Account
, but CPU limit issues usually run deeper than a simple nested loop or two.
About the only answer I can give you here is that you need to spend a fair amount of time profiling your code so you can accurately characterize the overall performance, and identify the problem areas (which methods are being called most often? which methods consume the most CPU time/queries/etc...?)
Turning up the debug log levels for the Apex category, and making use of the "Analysis" perspective in the dev console (With a debug log open in the dev console: Test -> Switch Perspective -> Analysis) will give you additional buttons and panels to play around with that should help you gather more information.
add a comment |
Nested loops are not inherently evil, and are required if you're working on a nested structure like this.
You could rewrite this particular query to be on Opportunity
instead of Account
, but CPU limit issues usually run deeper than a simple nested loop or two.
About the only answer I can give you here is that you need to spend a fair amount of time profiling your code so you can accurately characterize the overall performance, and identify the problem areas (which methods are being called most often? which methods consume the most CPU time/queries/etc...?)
Turning up the debug log levels for the Apex category, and making use of the "Analysis" perspective in the dev console (With a debug log open in the dev console: Test -> Switch Perspective -> Analysis) will give you additional buttons and panels to play around with that should help you gather more information.
add a comment |
Nested loops are not inherently evil, and are required if you're working on a nested structure like this.
You could rewrite this particular query to be on Opportunity
instead of Account
, but CPU limit issues usually run deeper than a simple nested loop or two.
About the only answer I can give you here is that you need to spend a fair amount of time profiling your code so you can accurately characterize the overall performance, and identify the problem areas (which methods are being called most often? which methods consume the most CPU time/queries/etc...?)
Turning up the debug log levels for the Apex category, and making use of the "Analysis" perspective in the dev console (With a debug log open in the dev console: Test -> Switch Perspective -> Analysis) will give you additional buttons and panels to play around with that should help you gather more information.
Nested loops are not inherently evil, and are required if you're working on a nested structure like this.
You could rewrite this particular query to be on Opportunity
instead of Account
, but CPU limit issues usually run deeper than a simple nested loop or two.
About the only answer I can give you here is that you need to spend a fair amount of time profiling your code so you can accurately characterize the overall performance, and identify the problem areas (which methods are being called most often? which methods consume the most CPU time/queries/etc...?)
Turning up the debug log levels for the Apex category, and making use of the "Analysis" perspective in the dev console (With a debug log open in the dev console: Test -> Switch Perspective -> Analysis) will give you additional buttons and panels to play around with that should help you gather more information.
answered Feb 14 at 19:19
Derek FDerek F
21.1k52353
21.1k52353
add a comment |
add a comment |
You could try querying the Opportunity table like so.
List<Opportunity> opp = [Select Id From Opportunity Where
AccountId IN (Select Id From Account Where Id = :accountIds)];
Otherwise if you are running into heap errors you might need to optimize your code before and after the query or move your code into batch.
add a comment |
You could try querying the Opportunity table like so.
List<Opportunity> opp = [Select Id From Opportunity Where
AccountId IN (Select Id From Account Where Id = :accountIds)];
Otherwise if you are running into heap errors you might need to optimize your code before and after the query or move your code into batch.
add a comment |
You could try querying the Opportunity table like so.
List<Opportunity> opp = [Select Id From Opportunity Where
AccountId IN (Select Id From Account Where Id = :accountIds)];
Otherwise if you are running into heap errors you might need to optimize your code before and after the query or move your code into batch.
You could try querying the Opportunity table like so.
List<Opportunity> opp = [Select Id From Opportunity Where
AccountId IN (Select Id From Account Where Id = :accountIds)];
Otherwise if you are running into heap errors you might need to optimize your code before and after the query or move your code into batch.
edited Feb 14 at 21:43
answered Feb 14 at 19:22


joshiijoshii
949
949
add a comment |
add a comment |
Thanks for contributing an answer to Salesforce Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsalesforce.stackexchange.com%2fquestions%2f250387%2fhow-can-i-efficiently-loop-through-opportunities-on-a-list-of-accounts%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
f AB2trLA68FzdokLzxJqeSoyJsRa,oPIfP