Are std::tuple and std::tuple considered same type by std::vector?
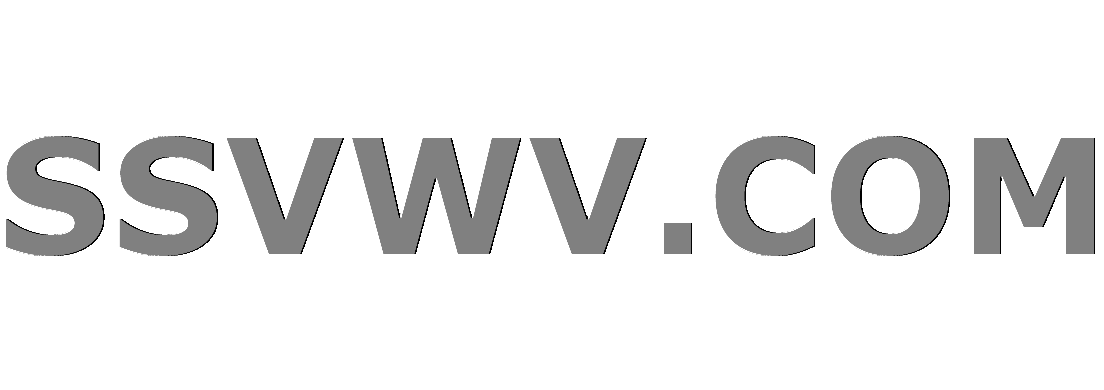
Multi tool use
I have a variable defined like this
auto drum = std::make_tuple
( std::make_tuple
( 0.3f
, ExampleClass
, (ExampleClass& instance) {return instance.eGetter ();}
)
);
I expect drum
to be a tuple of tuple. (i.e. ((a, b, c))
).
And I have another variable defined like this
auto base = std::make_tuple
( 0.48f
, ExampleClass
, (ExampleClass& instance) {return instance.eGetter ();}
);
which I expect to be just a tuple of three elements (i.e (a, b, c)
)
I also have a vector defined as follows
std::vector<std::tuple<std::tuple< float
, ExampleClass
, std::function<float (ExampleClass&)>
>>> listOfInstruments;
Now if I add drum
to listOfInstruments
I expect no errors.
Which was indeed the case with listOfInstruments.push_back(drum);
Where I expected an error was here listOfInstuments.push_back(base);
but the code compiles just fine.
Since listOfInstruments
has type 'tuple of tuples', should't adding just 'tuple' cause some error? Unless both ()
and (())
are considered same types by std::vector
. Or am I completely wrong and there's something else at work here?
Can't seem to figure it out.
c++ tuples
add a comment |
I have a variable defined like this
auto drum = std::make_tuple
( std::make_tuple
( 0.3f
, ExampleClass
, (ExampleClass& instance) {return instance.eGetter ();}
)
);
I expect drum
to be a tuple of tuple. (i.e. ((a, b, c))
).
And I have another variable defined like this
auto base = std::make_tuple
( 0.48f
, ExampleClass
, (ExampleClass& instance) {return instance.eGetter ();}
);
which I expect to be just a tuple of three elements (i.e (a, b, c)
)
I also have a vector defined as follows
std::vector<std::tuple<std::tuple< float
, ExampleClass
, std::function<float (ExampleClass&)>
>>> listOfInstruments;
Now if I add drum
to listOfInstruments
I expect no errors.
Which was indeed the case with listOfInstruments.push_back(drum);
Where I expected an error was here listOfInstuments.push_back(base);
but the code compiles just fine.
Since listOfInstruments
has type 'tuple of tuples', should't adding just 'tuple' cause some error? Unless both ()
and (())
are considered same types by std::vector
. Or am I completely wrong and there's something else at work here?
Can't seem to figure it out.
c++ tuples
1
As an aside, I'd strongly discourage you from declaring astd::tuple
of one element, and instead use the element type.
– Caleth
Jan 22 at 12:15
add a comment |
I have a variable defined like this
auto drum = std::make_tuple
( std::make_tuple
( 0.3f
, ExampleClass
, (ExampleClass& instance) {return instance.eGetter ();}
)
);
I expect drum
to be a tuple of tuple. (i.e. ((a, b, c))
).
And I have another variable defined like this
auto base = std::make_tuple
( 0.48f
, ExampleClass
, (ExampleClass& instance) {return instance.eGetter ();}
);
which I expect to be just a tuple of three elements (i.e (a, b, c)
)
I also have a vector defined as follows
std::vector<std::tuple<std::tuple< float
, ExampleClass
, std::function<float (ExampleClass&)>
>>> listOfInstruments;
Now if I add drum
to listOfInstruments
I expect no errors.
Which was indeed the case with listOfInstruments.push_back(drum);
Where I expected an error was here listOfInstuments.push_back(base);
but the code compiles just fine.
Since listOfInstruments
has type 'tuple of tuples', should't adding just 'tuple' cause some error? Unless both ()
and (())
are considered same types by std::vector
. Or am I completely wrong and there's something else at work here?
Can't seem to figure it out.
c++ tuples
I have a variable defined like this
auto drum = std::make_tuple
( std::make_tuple
( 0.3f
, ExampleClass
, (ExampleClass& instance) {return instance.eGetter ();}
)
);
I expect drum
to be a tuple of tuple. (i.e. ((a, b, c))
).
And I have another variable defined like this
auto base = std::make_tuple
( 0.48f
, ExampleClass
, (ExampleClass& instance) {return instance.eGetter ();}
);
which I expect to be just a tuple of three elements (i.e (a, b, c)
)
I also have a vector defined as follows
std::vector<std::tuple<std::tuple< float
, ExampleClass
, std::function<float (ExampleClass&)>
>>> listOfInstruments;
Now if I add drum
to listOfInstruments
I expect no errors.
Which was indeed the case with listOfInstruments.push_back(drum);
Where I expected an error was here listOfInstuments.push_back(base);
but the code compiles just fine.
Since listOfInstruments
has type 'tuple of tuples', should't adding just 'tuple' cause some error? Unless both ()
and (())
are considered same types by std::vector
. Or am I completely wrong and there's something else at work here?
Can't seem to figure it out.
c++ tuples
c++ tuples
asked Jan 22 at 10:30
atisatis
386212
386212
1
As an aside, I'd strongly discourage you from declaring astd::tuple
of one element, and instead use the element type.
– Caleth
Jan 22 at 12:15
add a comment |
1
As an aside, I'd strongly discourage you from declaring astd::tuple
of one element, and instead use the element type.
– Caleth
Jan 22 at 12:15
1
1
As an aside, I'd strongly discourage you from declaring a
std::tuple
of one element, and instead use the element type.– Caleth
Jan 22 at 12:15
As an aside, I'd strongly discourage you from declaring a
std::tuple
of one element, and instead use the element type.– Caleth
Jan 22 at 12:15
add a comment |
1 Answer
1
active
oldest
votes
Tuples and vectors are mostly red herrings here. The way that works is simply that push_back
, like any function, can perform implicit conversions on its argument, as demonstrated by the following working snippet:
#include <vector>
struct A { };
struct B {
B(A const &) { }
};
int main() {
std::vector<B> v;
v.push_back(A{});
}
Going back to tuple, we can see that it has (among others) a conditionally-explicit constructor (#2 here) which takes references to the tuple's members-to-be:
tuple( const Types&... args );
This constructor is implicit if and only if all of the members have implicit copy constructors, which is the case here (as synthesized constructors are implicit indeed). This means that std::tuple<...>
is implicitly convertible to std::tuple<std::tuple<...>>
, which is what you're observing.
1
That constructor is "conditionally explicit"; you might want to explain why it is implicit in this case.
– Yakk - Adam Nevraumont
Jan 22 at 15:22
2
@Yakk-AdamNevraumont uuuh... I had missed that note, but to be fair I don't understand why that is myself.
– Quentin
Jan 22 at 15:53
Perhaps a link to this question about conditionally explicit constructors is helpful.
– Daniel Jour
Jan 22 at 18:15
@DanielJour that's how one would go and implement such a constructor, but I don't get what types could possibly failstd::is_convertible<const Ti&, Ti>
and why it would make sense for the constructor to be explicit in that case...
– Quentin
Jan 22 at 18:17
1
@Quentin I believe that would fail ifTi
's copy constructor were explicit. So that constructor is explicit if any of its members' copy constructors are explicit.
– David Brown
Jan 22 at 18:58
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54306241%2fare-stdtuple-and-stdtuplestdtuple-considered-same-type-by-stdvector%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Tuples and vectors are mostly red herrings here. The way that works is simply that push_back
, like any function, can perform implicit conversions on its argument, as demonstrated by the following working snippet:
#include <vector>
struct A { };
struct B {
B(A const &) { }
};
int main() {
std::vector<B> v;
v.push_back(A{});
}
Going back to tuple, we can see that it has (among others) a conditionally-explicit constructor (#2 here) which takes references to the tuple's members-to-be:
tuple( const Types&... args );
This constructor is implicit if and only if all of the members have implicit copy constructors, which is the case here (as synthesized constructors are implicit indeed). This means that std::tuple<...>
is implicitly convertible to std::tuple<std::tuple<...>>
, which is what you're observing.
1
That constructor is "conditionally explicit"; you might want to explain why it is implicit in this case.
– Yakk - Adam Nevraumont
Jan 22 at 15:22
2
@Yakk-AdamNevraumont uuuh... I had missed that note, but to be fair I don't understand why that is myself.
– Quentin
Jan 22 at 15:53
Perhaps a link to this question about conditionally explicit constructors is helpful.
– Daniel Jour
Jan 22 at 18:15
@DanielJour that's how one would go and implement such a constructor, but I don't get what types could possibly failstd::is_convertible<const Ti&, Ti>
and why it would make sense for the constructor to be explicit in that case...
– Quentin
Jan 22 at 18:17
1
@Quentin I believe that would fail ifTi
's copy constructor were explicit. So that constructor is explicit if any of its members' copy constructors are explicit.
– David Brown
Jan 22 at 18:58
|
show 1 more comment
Tuples and vectors are mostly red herrings here. The way that works is simply that push_back
, like any function, can perform implicit conversions on its argument, as demonstrated by the following working snippet:
#include <vector>
struct A { };
struct B {
B(A const &) { }
};
int main() {
std::vector<B> v;
v.push_back(A{});
}
Going back to tuple, we can see that it has (among others) a conditionally-explicit constructor (#2 here) which takes references to the tuple's members-to-be:
tuple( const Types&... args );
This constructor is implicit if and only if all of the members have implicit copy constructors, which is the case here (as synthesized constructors are implicit indeed). This means that std::tuple<...>
is implicitly convertible to std::tuple<std::tuple<...>>
, which is what you're observing.
1
That constructor is "conditionally explicit"; you might want to explain why it is implicit in this case.
– Yakk - Adam Nevraumont
Jan 22 at 15:22
2
@Yakk-AdamNevraumont uuuh... I had missed that note, but to be fair I don't understand why that is myself.
– Quentin
Jan 22 at 15:53
Perhaps a link to this question about conditionally explicit constructors is helpful.
– Daniel Jour
Jan 22 at 18:15
@DanielJour that's how one would go and implement such a constructor, but I don't get what types could possibly failstd::is_convertible<const Ti&, Ti>
and why it would make sense for the constructor to be explicit in that case...
– Quentin
Jan 22 at 18:17
1
@Quentin I believe that would fail ifTi
's copy constructor were explicit. So that constructor is explicit if any of its members' copy constructors are explicit.
– David Brown
Jan 22 at 18:58
|
show 1 more comment
Tuples and vectors are mostly red herrings here. The way that works is simply that push_back
, like any function, can perform implicit conversions on its argument, as demonstrated by the following working snippet:
#include <vector>
struct A { };
struct B {
B(A const &) { }
};
int main() {
std::vector<B> v;
v.push_back(A{});
}
Going back to tuple, we can see that it has (among others) a conditionally-explicit constructor (#2 here) which takes references to the tuple's members-to-be:
tuple( const Types&... args );
This constructor is implicit if and only if all of the members have implicit copy constructors, which is the case here (as synthesized constructors are implicit indeed). This means that std::tuple<...>
is implicitly convertible to std::tuple<std::tuple<...>>
, which is what you're observing.
Tuples and vectors are mostly red herrings here. The way that works is simply that push_back
, like any function, can perform implicit conversions on its argument, as demonstrated by the following working snippet:
#include <vector>
struct A { };
struct B {
B(A const &) { }
};
int main() {
std::vector<B> v;
v.push_back(A{});
}
Going back to tuple, we can see that it has (among others) a conditionally-explicit constructor (#2 here) which takes references to the tuple's members-to-be:
tuple( const Types&... args );
This constructor is implicit if and only if all of the members have implicit copy constructors, which is the case here (as synthesized constructors are implicit indeed). This means that std::tuple<...>
is implicitly convertible to std::tuple<std::tuple<...>>
, which is what you're observing.
edited Jan 22 at 19:02
answered Jan 22 at 10:36
QuentinQuentin
46.3k589143
46.3k589143
1
That constructor is "conditionally explicit"; you might want to explain why it is implicit in this case.
– Yakk - Adam Nevraumont
Jan 22 at 15:22
2
@Yakk-AdamNevraumont uuuh... I had missed that note, but to be fair I don't understand why that is myself.
– Quentin
Jan 22 at 15:53
Perhaps a link to this question about conditionally explicit constructors is helpful.
– Daniel Jour
Jan 22 at 18:15
@DanielJour that's how one would go and implement such a constructor, but I don't get what types could possibly failstd::is_convertible<const Ti&, Ti>
and why it would make sense for the constructor to be explicit in that case...
– Quentin
Jan 22 at 18:17
1
@Quentin I believe that would fail ifTi
's copy constructor were explicit. So that constructor is explicit if any of its members' copy constructors are explicit.
– David Brown
Jan 22 at 18:58
|
show 1 more comment
1
That constructor is "conditionally explicit"; you might want to explain why it is implicit in this case.
– Yakk - Adam Nevraumont
Jan 22 at 15:22
2
@Yakk-AdamNevraumont uuuh... I had missed that note, but to be fair I don't understand why that is myself.
– Quentin
Jan 22 at 15:53
Perhaps a link to this question about conditionally explicit constructors is helpful.
– Daniel Jour
Jan 22 at 18:15
@DanielJour that's how one would go and implement such a constructor, but I don't get what types could possibly failstd::is_convertible<const Ti&, Ti>
and why it would make sense for the constructor to be explicit in that case...
– Quentin
Jan 22 at 18:17
1
@Quentin I believe that would fail ifTi
's copy constructor were explicit. So that constructor is explicit if any of its members' copy constructors are explicit.
– David Brown
Jan 22 at 18:58
1
1
That constructor is "conditionally explicit"; you might want to explain why it is implicit in this case.
– Yakk - Adam Nevraumont
Jan 22 at 15:22
That constructor is "conditionally explicit"; you might want to explain why it is implicit in this case.
– Yakk - Adam Nevraumont
Jan 22 at 15:22
2
2
@Yakk-AdamNevraumont uuuh... I had missed that note, but to be fair I don't understand why that is myself.
– Quentin
Jan 22 at 15:53
@Yakk-AdamNevraumont uuuh... I had missed that note, but to be fair I don't understand why that is myself.
– Quentin
Jan 22 at 15:53
Perhaps a link to this question about conditionally explicit constructors is helpful.
– Daniel Jour
Jan 22 at 18:15
Perhaps a link to this question about conditionally explicit constructors is helpful.
– Daniel Jour
Jan 22 at 18:15
@DanielJour that's how one would go and implement such a constructor, but I don't get what types could possibly fail
std::is_convertible<const Ti&, Ti>
and why it would make sense for the constructor to be explicit in that case...– Quentin
Jan 22 at 18:17
@DanielJour that's how one would go and implement such a constructor, but I don't get what types could possibly fail
std::is_convertible<const Ti&, Ti>
and why it would make sense for the constructor to be explicit in that case...– Quentin
Jan 22 at 18:17
1
1
@Quentin I believe that would fail if
Ti
's copy constructor were explicit. So that constructor is explicit if any of its members' copy constructors are explicit.– David Brown
Jan 22 at 18:58
@Quentin I believe that would fail if
Ti
's copy constructor were explicit. So that constructor is explicit if any of its members' copy constructors are explicit.– David Brown
Jan 22 at 18:58
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54306241%2fare-stdtuple-and-stdtuplestdtuple-considered-same-type-by-stdvector%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1yZUVAu NfzGLCPcAG4HPmDiSUPnK67M ESW,T5cjWRPZ67B34z g pFWg0dS1
1
As an aside, I'd strongly discourage you from declaring a
std::tuple
of one element, and instead use the element type.– Caleth
Jan 22 at 12:15