Difference between “new Foo()” and “&Foo()” as parameters
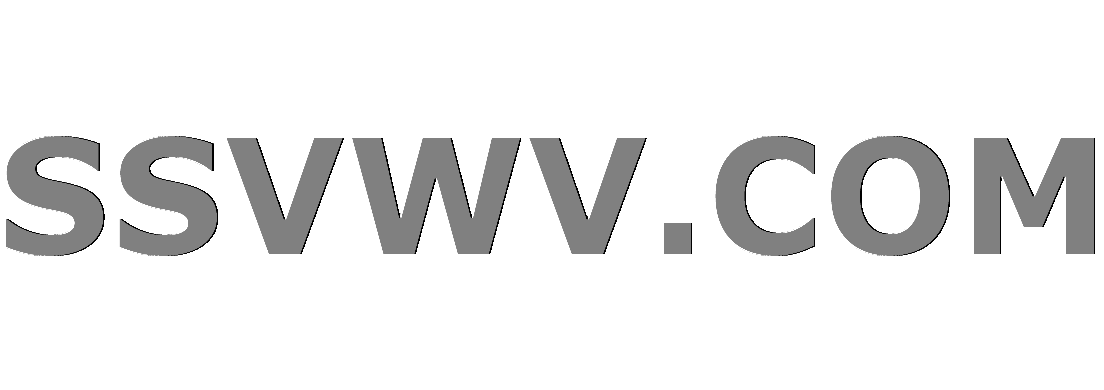
Multi tool use
I have some questions concerning the difference between the keyword new
and &
in a certain context.
Let’s say this is my code:
struct Base {};
struct Foo : Base {};
struct Storage
{
void save(Base * object) {}
Base * content;
};
int main()
{
Storage s1, s2;
s1.save(new Foo());
s2.save(&Foo());
}
After the execution of main, s1
will hold a pointer to an object of type Foo
. Yet s2
will hold a pointer to an object of type Base
. s2.content
will only point towards an object of type Foo
until the save method has finished execution.
Please correct me if I am wrong:
As far as I understand new Foo()
creates a pointer to a new object of type Foo
. &Foo()
on the other hand first creates a new object of type Foo
and then points to it.
What exactly is the difference between new Foo()
and &Foo()
then? Obviously both give you a pointer to an existing object of type Foo
.
Why does the object created by new Foo()
persist after execution of the save method whereas the object created via &Foo()
does not?
May it be that &Foo()
creates a temporary object, which will cease to exist after the execution of save? If yes, how can I prolong the life of the object created via &Foo()
to make it live (at least) until the destruction of s2
?
Edit 1:
Thank you very much for the quick answers! I'm simply using Visual Studio, so maybe &Foo()
compiling is some Microsoft specific stuff...
c++ reference parameter-passing new-operator
add a comment |
I have some questions concerning the difference between the keyword new
and &
in a certain context.
Let’s say this is my code:
struct Base {};
struct Foo : Base {};
struct Storage
{
void save(Base * object) {}
Base * content;
};
int main()
{
Storage s1, s2;
s1.save(new Foo());
s2.save(&Foo());
}
After the execution of main, s1
will hold a pointer to an object of type Foo
. Yet s2
will hold a pointer to an object of type Base
. s2.content
will only point towards an object of type Foo
until the save method has finished execution.
Please correct me if I am wrong:
As far as I understand new Foo()
creates a pointer to a new object of type Foo
. &Foo()
on the other hand first creates a new object of type Foo
and then points to it.
What exactly is the difference between new Foo()
and &Foo()
then? Obviously both give you a pointer to an existing object of type Foo
.
Why does the object created by new Foo()
persist after execution of the save method whereas the object created via &Foo()
does not?
May it be that &Foo()
creates a temporary object, which will cease to exist after the execution of save? If yes, how can I prolong the life of the object created via &Foo()
to make it live (at least) until the destruction of s2
?
Edit 1:
Thank you very much for the quick answers! I'm simply using Visual Studio, so maybe &Foo()
compiling is some Microsoft specific stuff...
c++ reference parameter-passing new-operator
1
You can't prolong the life of &Foo()
– drescherjm
Dec 30 '18 at 15:20
6
The most obvious difference would be that one compiles while the other doesn't... wandbox.org/permlink/qWn4eVLP3HWhIeGt
– Baum mit Augen
Dec 30 '18 at 15:20
1
"how can I prolong the life of the object created via "&Foo()" ... that's whatnew Foo()
is for.
– Eljay
Dec 30 '18 at 15:33
"Yets2
will hold a pointer to an object of typeBase
." - what makes you think that?
– Bergi
Dec 30 '18 at 20:10
If you increased the warning level to at least 4, you'd get "warning C4238: nonstandard extension used: class rvalue used as lvalue".
– isanae
Dec 30 '18 at 22:03
add a comment |
I have some questions concerning the difference between the keyword new
and &
in a certain context.
Let’s say this is my code:
struct Base {};
struct Foo : Base {};
struct Storage
{
void save(Base * object) {}
Base * content;
};
int main()
{
Storage s1, s2;
s1.save(new Foo());
s2.save(&Foo());
}
After the execution of main, s1
will hold a pointer to an object of type Foo
. Yet s2
will hold a pointer to an object of type Base
. s2.content
will only point towards an object of type Foo
until the save method has finished execution.
Please correct me if I am wrong:
As far as I understand new Foo()
creates a pointer to a new object of type Foo
. &Foo()
on the other hand first creates a new object of type Foo
and then points to it.
What exactly is the difference between new Foo()
and &Foo()
then? Obviously both give you a pointer to an existing object of type Foo
.
Why does the object created by new Foo()
persist after execution of the save method whereas the object created via &Foo()
does not?
May it be that &Foo()
creates a temporary object, which will cease to exist after the execution of save? If yes, how can I prolong the life of the object created via &Foo()
to make it live (at least) until the destruction of s2
?
Edit 1:
Thank you very much for the quick answers! I'm simply using Visual Studio, so maybe &Foo()
compiling is some Microsoft specific stuff...
c++ reference parameter-passing new-operator
I have some questions concerning the difference between the keyword new
and &
in a certain context.
Let’s say this is my code:
struct Base {};
struct Foo : Base {};
struct Storage
{
void save(Base * object) {}
Base * content;
};
int main()
{
Storage s1, s2;
s1.save(new Foo());
s2.save(&Foo());
}
After the execution of main, s1
will hold a pointer to an object of type Foo
. Yet s2
will hold a pointer to an object of type Base
. s2.content
will only point towards an object of type Foo
until the save method has finished execution.
Please correct me if I am wrong:
As far as I understand new Foo()
creates a pointer to a new object of type Foo
. &Foo()
on the other hand first creates a new object of type Foo
and then points to it.
What exactly is the difference between new Foo()
and &Foo()
then? Obviously both give you a pointer to an existing object of type Foo
.
Why does the object created by new Foo()
persist after execution of the save method whereas the object created via &Foo()
does not?
May it be that &Foo()
creates a temporary object, which will cease to exist after the execution of save? If yes, how can I prolong the life of the object created via &Foo()
to make it live (at least) until the destruction of s2
?
Edit 1:
Thank you very much for the quick answers! I'm simply using Visual Studio, so maybe &Foo()
compiling is some Microsoft specific stuff...
c++ reference parameter-passing new-operator
c++ reference parameter-passing new-operator
edited Dec 30 '18 at 22:01


isanae
2,50111336
2,50111336
asked Dec 30 '18 at 15:15


Domi P.Domi P.
786
786
1
You can't prolong the life of &Foo()
– drescherjm
Dec 30 '18 at 15:20
6
The most obvious difference would be that one compiles while the other doesn't... wandbox.org/permlink/qWn4eVLP3HWhIeGt
– Baum mit Augen
Dec 30 '18 at 15:20
1
"how can I prolong the life of the object created via "&Foo()" ... that's whatnew Foo()
is for.
– Eljay
Dec 30 '18 at 15:33
"Yets2
will hold a pointer to an object of typeBase
." - what makes you think that?
– Bergi
Dec 30 '18 at 20:10
If you increased the warning level to at least 4, you'd get "warning C4238: nonstandard extension used: class rvalue used as lvalue".
– isanae
Dec 30 '18 at 22:03
add a comment |
1
You can't prolong the life of &Foo()
– drescherjm
Dec 30 '18 at 15:20
6
The most obvious difference would be that one compiles while the other doesn't... wandbox.org/permlink/qWn4eVLP3HWhIeGt
– Baum mit Augen
Dec 30 '18 at 15:20
1
"how can I prolong the life of the object created via "&Foo()" ... that's whatnew Foo()
is for.
– Eljay
Dec 30 '18 at 15:33
"Yets2
will hold a pointer to an object of typeBase
." - what makes you think that?
– Bergi
Dec 30 '18 at 20:10
If you increased the warning level to at least 4, you'd get "warning C4238: nonstandard extension used: class rvalue used as lvalue".
– isanae
Dec 30 '18 at 22:03
1
1
You can't prolong the life of &Foo()
– drescherjm
Dec 30 '18 at 15:20
You can't prolong the life of &Foo()
– drescherjm
Dec 30 '18 at 15:20
6
6
The most obvious difference would be that one compiles while the other doesn't... wandbox.org/permlink/qWn4eVLP3HWhIeGt
– Baum mit Augen
Dec 30 '18 at 15:20
The most obvious difference would be that one compiles while the other doesn't... wandbox.org/permlink/qWn4eVLP3HWhIeGt
– Baum mit Augen
Dec 30 '18 at 15:20
1
1
"how can I prolong the life of the object created via "&Foo()" ... that's what
new Foo()
is for.– Eljay
Dec 30 '18 at 15:33
"how can I prolong the life of the object created via "&Foo()" ... that's what
new Foo()
is for.– Eljay
Dec 30 '18 at 15:33
"Yet
s2
will hold a pointer to an object of type Base
." - what makes you think that?– Bergi
Dec 30 '18 at 20:10
"Yet
s2
will hold a pointer to an object of type Base
." - what makes you think that?– Bergi
Dec 30 '18 at 20:10
If you increased the warning level to at least 4, you'd get "warning C4238: nonstandard extension used: class rvalue used as lvalue".
– isanae
Dec 30 '18 at 22:03
If you increased the warning level to at least 4, you'd get "warning C4238: nonstandard extension used: class rvalue used as lvalue".
– isanae
Dec 30 '18 at 22:03
add a comment |
2 Answers
2
active
oldest
votes
What exactly is the difference between "
new Foo()
" and "&Foo()
" then?
Obviously both give you a pointer to an existing object of type Foo.
Why does the object created by "
new Foo()
" persist after execution of
the save method whereas the object created via "&Foo()
" does not?
new Foo()
new Foo();
This creates a dynamically-allocated Foo
object and returns a pointer pointing to that object. Dynamically-allocated objects will persist until they're explicitly deleted by the programmer:
Foo* foo = new Foo();
delete foo; // delete the object.
&Foo()
Foo();
This creates a Foo
object using automatic-storage. This means its lifetime, when the object is deleted, is decided by the scope that the object lives in:
{
Foo foo{}; // foo lives in automatic storage.
} // end of scope, foo dies
In your case you are creating a new Foo
object and you're passing the address of this anonymous object to Storage::save
. This object will be destroyed at the end of the full expression. This basically means after s2.save()
returns your object will be destroyed and the pointer pointing to it in s2
will be dangling and dereferencing it will be undefined behaviour.
If yes, how can I prolong the life of the object created via "&Foo()"
to make it live (at least) until the destruction of s2?
You can't. You probably want a smart pointer here such as std::unique_ptr
.
Note that taking the address of a temporary is non-standard and thus this code is non-compliant to begin with. Your compiler is probably using an extension to allow it. MSVC is known for allowing this.
add a comment |
The expression Foo()
creates a new temporary object, and using the address-of operator &
on that temporary object will result in a compiler error since it's not allowed to get the address of temporary objects like that (Foo()
is an rvalue and the address-of operator can't be used on those).
With new Foo
you create a non temporary object, and the result is a pointer to that object. The life-time of this object is until you explicitly delete
it. And if you don't delete
it then you will have a memory leak.
2
VS allows the binding for some reason
– Lightness Races in Orbit
Dec 30 '18 at 17:21
@LightnessRacesinOrbit/W4
will warn about it. It's unfortunate that/permissive-
doesn't make it a hard error though.
– isanae
Dec 30 '18 at 22:04
@LightnessRacesinOrbit It's a very non-standard extension of MSVC++, unfortunately.
– Some programmer dude
Dec 30 '18 at 22:11
You are both correct.
– Lightness Races in Orbit
Dec 31 '18 at 0:34
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53978791%2fdifference-between-new-foo-and-foo-as-parameters%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
What exactly is the difference between "
new Foo()
" and "&Foo()
" then?
Obviously both give you a pointer to an existing object of type Foo.
Why does the object created by "
new Foo()
" persist after execution of
the save method whereas the object created via "&Foo()
" does not?
new Foo()
new Foo();
This creates a dynamically-allocated Foo
object and returns a pointer pointing to that object. Dynamically-allocated objects will persist until they're explicitly deleted by the programmer:
Foo* foo = new Foo();
delete foo; // delete the object.
&Foo()
Foo();
This creates a Foo
object using automatic-storage. This means its lifetime, when the object is deleted, is decided by the scope that the object lives in:
{
Foo foo{}; // foo lives in automatic storage.
} // end of scope, foo dies
In your case you are creating a new Foo
object and you're passing the address of this anonymous object to Storage::save
. This object will be destroyed at the end of the full expression. This basically means after s2.save()
returns your object will be destroyed and the pointer pointing to it in s2
will be dangling and dereferencing it will be undefined behaviour.
If yes, how can I prolong the life of the object created via "&Foo()"
to make it live (at least) until the destruction of s2?
You can't. You probably want a smart pointer here such as std::unique_ptr
.
Note that taking the address of a temporary is non-standard and thus this code is non-compliant to begin with. Your compiler is probably using an extension to allow it. MSVC is known for allowing this.
add a comment |
What exactly is the difference between "
new Foo()
" and "&Foo()
" then?
Obviously both give you a pointer to an existing object of type Foo.
Why does the object created by "
new Foo()
" persist after execution of
the save method whereas the object created via "&Foo()
" does not?
new Foo()
new Foo();
This creates a dynamically-allocated Foo
object and returns a pointer pointing to that object. Dynamically-allocated objects will persist until they're explicitly deleted by the programmer:
Foo* foo = new Foo();
delete foo; // delete the object.
&Foo()
Foo();
This creates a Foo
object using automatic-storage. This means its lifetime, when the object is deleted, is decided by the scope that the object lives in:
{
Foo foo{}; // foo lives in automatic storage.
} // end of scope, foo dies
In your case you are creating a new Foo
object and you're passing the address of this anonymous object to Storage::save
. This object will be destroyed at the end of the full expression. This basically means after s2.save()
returns your object will be destroyed and the pointer pointing to it in s2
will be dangling and dereferencing it will be undefined behaviour.
If yes, how can I prolong the life of the object created via "&Foo()"
to make it live (at least) until the destruction of s2?
You can't. You probably want a smart pointer here such as std::unique_ptr
.
Note that taking the address of a temporary is non-standard and thus this code is non-compliant to begin with. Your compiler is probably using an extension to allow it. MSVC is known for allowing this.
add a comment |
What exactly is the difference between "
new Foo()
" and "&Foo()
" then?
Obviously both give you a pointer to an existing object of type Foo.
Why does the object created by "
new Foo()
" persist after execution of
the save method whereas the object created via "&Foo()
" does not?
new Foo()
new Foo();
This creates a dynamically-allocated Foo
object and returns a pointer pointing to that object. Dynamically-allocated objects will persist until they're explicitly deleted by the programmer:
Foo* foo = new Foo();
delete foo; // delete the object.
&Foo()
Foo();
This creates a Foo
object using automatic-storage. This means its lifetime, when the object is deleted, is decided by the scope that the object lives in:
{
Foo foo{}; // foo lives in automatic storage.
} // end of scope, foo dies
In your case you are creating a new Foo
object and you're passing the address of this anonymous object to Storage::save
. This object will be destroyed at the end of the full expression. This basically means after s2.save()
returns your object will be destroyed and the pointer pointing to it in s2
will be dangling and dereferencing it will be undefined behaviour.
If yes, how can I prolong the life of the object created via "&Foo()"
to make it live (at least) until the destruction of s2?
You can't. You probably want a smart pointer here such as std::unique_ptr
.
Note that taking the address of a temporary is non-standard and thus this code is non-compliant to begin with. Your compiler is probably using an extension to allow it. MSVC is known for allowing this.
What exactly is the difference between "
new Foo()
" and "&Foo()
" then?
Obviously both give you a pointer to an existing object of type Foo.
Why does the object created by "
new Foo()
" persist after execution of
the save method whereas the object created via "&Foo()
" does not?
new Foo()
new Foo();
This creates a dynamically-allocated Foo
object and returns a pointer pointing to that object. Dynamically-allocated objects will persist until they're explicitly deleted by the programmer:
Foo* foo = new Foo();
delete foo; // delete the object.
&Foo()
Foo();
This creates a Foo
object using automatic-storage. This means its lifetime, when the object is deleted, is decided by the scope that the object lives in:
{
Foo foo{}; // foo lives in automatic storage.
} // end of scope, foo dies
In your case you are creating a new Foo
object and you're passing the address of this anonymous object to Storage::save
. This object will be destroyed at the end of the full expression. This basically means after s2.save()
returns your object will be destroyed and the pointer pointing to it in s2
will be dangling and dereferencing it will be undefined behaviour.
If yes, how can I prolong the life of the object created via "&Foo()"
to make it live (at least) until the destruction of s2?
You can't. You probably want a smart pointer here such as std::unique_ptr
.
Note that taking the address of a temporary is non-standard and thus this code is non-compliant to begin with. Your compiler is probably using an extension to allow it. MSVC is known for allowing this.
edited Dec 30 '18 at 15:26
answered Dec 30 '18 at 15:21


Sombrero ChickenSombrero Chicken
23.5k33077
23.5k33077
add a comment |
add a comment |
The expression Foo()
creates a new temporary object, and using the address-of operator &
on that temporary object will result in a compiler error since it's not allowed to get the address of temporary objects like that (Foo()
is an rvalue and the address-of operator can't be used on those).
With new Foo
you create a non temporary object, and the result is a pointer to that object. The life-time of this object is until you explicitly delete
it. And if you don't delete
it then you will have a memory leak.
2
VS allows the binding for some reason
– Lightness Races in Orbit
Dec 30 '18 at 17:21
@LightnessRacesinOrbit/W4
will warn about it. It's unfortunate that/permissive-
doesn't make it a hard error though.
– isanae
Dec 30 '18 at 22:04
@LightnessRacesinOrbit It's a very non-standard extension of MSVC++, unfortunately.
– Some programmer dude
Dec 30 '18 at 22:11
You are both correct.
– Lightness Races in Orbit
Dec 31 '18 at 0:34
add a comment |
The expression Foo()
creates a new temporary object, and using the address-of operator &
on that temporary object will result in a compiler error since it's not allowed to get the address of temporary objects like that (Foo()
is an rvalue and the address-of operator can't be used on those).
With new Foo
you create a non temporary object, and the result is a pointer to that object. The life-time of this object is until you explicitly delete
it. And if you don't delete
it then you will have a memory leak.
2
VS allows the binding for some reason
– Lightness Races in Orbit
Dec 30 '18 at 17:21
@LightnessRacesinOrbit/W4
will warn about it. It's unfortunate that/permissive-
doesn't make it a hard error though.
– isanae
Dec 30 '18 at 22:04
@LightnessRacesinOrbit It's a very non-standard extension of MSVC++, unfortunately.
– Some programmer dude
Dec 30 '18 at 22:11
You are both correct.
– Lightness Races in Orbit
Dec 31 '18 at 0:34
add a comment |
The expression Foo()
creates a new temporary object, and using the address-of operator &
on that temporary object will result in a compiler error since it's not allowed to get the address of temporary objects like that (Foo()
is an rvalue and the address-of operator can't be used on those).
With new Foo
you create a non temporary object, and the result is a pointer to that object. The life-time of this object is until you explicitly delete
it. And if you don't delete
it then you will have a memory leak.
The expression Foo()
creates a new temporary object, and using the address-of operator &
on that temporary object will result in a compiler error since it's not allowed to get the address of temporary objects like that (Foo()
is an rvalue and the address-of operator can't be used on those).
With new Foo
you create a non temporary object, and the result is a pointer to that object. The life-time of this object is until you explicitly delete
it. And if you don't delete
it then you will have a memory leak.
edited Dec 30 '18 at 15:21
answered Dec 30 '18 at 15:20


Some programmer dudeSome programmer dude
296k24250412
296k24250412
2
VS allows the binding for some reason
– Lightness Races in Orbit
Dec 30 '18 at 17:21
@LightnessRacesinOrbit/W4
will warn about it. It's unfortunate that/permissive-
doesn't make it a hard error though.
– isanae
Dec 30 '18 at 22:04
@LightnessRacesinOrbit It's a very non-standard extension of MSVC++, unfortunately.
– Some programmer dude
Dec 30 '18 at 22:11
You are both correct.
– Lightness Races in Orbit
Dec 31 '18 at 0:34
add a comment |
2
VS allows the binding for some reason
– Lightness Races in Orbit
Dec 30 '18 at 17:21
@LightnessRacesinOrbit/W4
will warn about it. It's unfortunate that/permissive-
doesn't make it a hard error though.
– isanae
Dec 30 '18 at 22:04
@LightnessRacesinOrbit It's a very non-standard extension of MSVC++, unfortunately.
– Some programmer dude
Dec 30 '18 at 22:11
You are both correct.
– Lightness Races in Orbit
Dec 31 '18 at 0:34
2
2
VS allows the binding for some reason
– Lightness Races in Orbit
Dec 30 '18 at 17:21
VS allows the binding for some reason
– Lightness Races in Orbit
Dec 30 '18 at 17:21
@LightnessRacesinOrbit
/W4
will warn about it. It's unfortunate that /permissive-
doesn't make it a hard error though.– isanae
Dec 30 '18 at 22:04
@LightnessRacesinOrbit
/W4
will warn about it. It's unfortunate that /permissive-
doesn't make it a hard error though.– isanae
Dec 30 '18 at 22:04
@LightnessRacesinOrbit It's a very non-standard extension of MSVC++, unfortunately.
– Some programmer dude
Dec 30 '18 at 22:11
@LightnessRacesinOrbit It's a very non-standard extension of MSVC++, unfortunately.
– Some programmer dude
Dec 30 '18 at 22:11
You are both correct.
– Lightness Races in Orbit
Dec 31 '18 at 0:34
You are both correct.
– Lightness Races in Orbit
Dec 31 '18 at 0:34
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53978791%2fdifference-between-new-foo-and-foo-as-parameters%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JFoeAuKyFu0yBQt,e0P7,ybClKXz5F8 P 0M,Snz,ML97 fagsTWjo8E8vzZBg,PiKw78
1
You can't prolong the life of &Foo()
– drescherjm
Dec 30 '18 at 15:20
6
The most obvious difference would be that one compiles while the other doesn't... wandbox.org/permlink/qWn4eVLP3HWhIeGt
– Baum mit Augen
Dec 30 '18 at 15:20
1
"how can I prolong the life of the object created via "&Foo()" ... that's what
new Foo()
is for.– Eljay
Dec 30 '18 at 15:33
"Yet
s2
will hold a pointer to an object of typeBase
." - what makes you think that?– Bergi
Dec 30 '18 at 20:10
If you increased the warning level to at least 4, you'd get "warning C4238: nonstandard extension used: class rvalue used as lvalue".
– isanae
Dec 30 '18 at 22:03